Calling block sequences (L SEQUENCE)
Block sequences are contiguous program parts (sequences) or single NC blocks in the current NC program or a global subroutine which can be executed once or several times with L SEQUENCE.
A bock sequence is defined by specifying the start and end identifications by:
- Block numbers N.. or
- Jump labels ([Stringlabel] analogous to the definition for $GOTO)
Notice
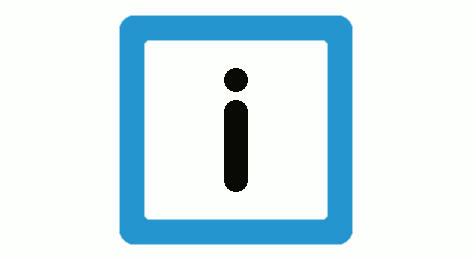
Every call of a block sequence is identical to a subroutine call. The same rules on nesting depth apply as for global subroutines.
Attention
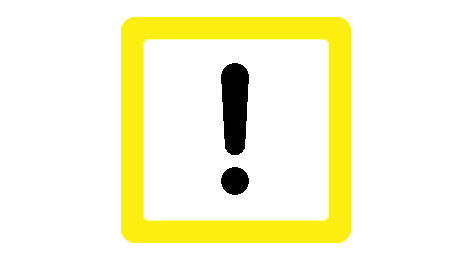
Context evaluation:
The program context in the subroutine is not set up until the first NC line of the block sequence is executed. All previous NC lines passed through are not evaluated. Previously defined variables/coordinate systems, parameters, modal statements, etc. are neither created nor initialised. Therefore, they are unknown or not available in the block sequence.
In particular when executing block sequences with control block statements ($IF-$ELSE-$ENDIF, $SWITCH,..), users must ensure themselves that they pass through the entry and return points without conflict.
Syntax of L SEQUENCE when using block numbers: |
L SEQUENCE [ [ NAME=<string> ] N.. [ N.. ] [ REPEAT=.. ] [ ENDTAG ] ] |
NAME=<string> | Name of the current subroutine or a global subroutine in which the block sequence makes a pass. Optional: If no name is programmed, the block sequence passes through the current NC program. |
N.. | Number of the first block to be executed (start number, start of block sequence) |
N.. | Number of the last block to be executed (return number, end of block sequence) Optionally, if both block numbers are identical, only this block is executed. |
REPEAT=.. | Number of repetitions of a block sequence, positive integer > 1. Optionally, if REPEAT is not specified, the block sequence makes a single pass. |
ENDTAG | Marks the call L SEQUENCE itself as an additional valid end of the block sequence. Optionally, if both ENDTAG and a return number N.. are programmed at the same time, the sequence end found first is the valid one. |
The controller searches for the programmed N (block) numbers in the specified NC program (which can also be the same program that calls up the command). The two N numbers mark the first and last NC blocks to be executed in the block sequence; NC blocks outside this block sequence are not executed.
It is recommended to use a unique ascending numbering format for the NC blocks.
The start and return numbers can also be swapped in the command when programmed. In the NC program, however, the block sequence always passes through from the lower N number to the higher N number.
An error message is issued if the start or return number is not found.
If the block sequence is to be executed multiple times (REPEAT > 1), the program starts at the start number again at the end of the block sequence. Once all passes have been executed, the program returns from the block sequence and the rest of the program sequence is continued.
If only an N number was specified in the command, only this line is passed. This corresponds to a call with two identical N numbers.
The L SEQUENCE call itself may be located in the block sequence defined by the N numbers. When the same call is read again, the following two reactions are possible:
Without ENDTAG the recall is ignored and the block sequence is executed until the return number.
With ENDTAG the L SEQUENCE call is marked as the valid sequence end and the block sequence is terminated.
Programing Example
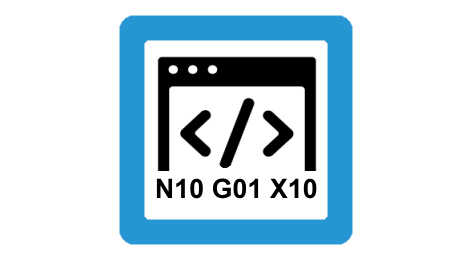
Calling block sequences with block numbers (L SEQUENCE)
Repeat block sequence between 2 block numbers once:
...
N20 ... ;Start number
...
N50 ... ;Return number
...
N80 L SEQUENCE [N20 N50] ; or..
N80 L SEQUENCE [N20 N50 REPEAT=1]
...
Repeat block sequence between 2 block numbers several times:
...
N20 ... ;Start number
...
N50 ... ;Return number
...
N80 L SEQUENCE [N20 N50 REPEAT=4]
...
Block sequence between 2 block numbers. Bracketed sequence call is ignored when the block sequence is executed since ENDTAG is not set:
...
N20 ... ;Start number
...
N40 L SEQUENCE [N20 N80]
...
N80 ... ;Return number
...
Block sequence between 2 block numbers. Bracketed sequence call is the first block sequence end found since ENDTAG is set:
...
N20 ... ;Start number
...
N40 L SEQUENCE [N20 N80 ENDTAG]
...
N80 ... ;Return number
...
Block sequence between 2 block numbers with ENDTAG. ENDTAG is not relevant since the return number is before the sequence call:
...
N20 ... ;Start number
...
N50 ... ;Return number
...
N80 L SEQUENCE [N20 N50 REPEAT=4 ENDTAG]
...
Repeat a single NC block several times:
...
N20 ... ;Start number
...
N80 L SEQUENCE [N20 REPEAT=4] ; or..
N80 L SEQUENCE [N20 N20 REPEAT=4]
...
Block sequence between 1 block number and ENDTAG:
...
N20 ... ;Start number
...
N80 L SEQUENCE [N20 ENDTAG]
...
Repeat block sequence between 2 block numbers several times. Sequence call is before the block sequence:
...
N80 L SEQUENCE [N100 N150 REPEAT=4]
...
N100 ... ;Start number
...
N150 ... ;Return number
Nested multiple call of block sequences between block numbers:
...
N40 L SEQUENCE [N60 N150 REPEAT=2] ;Sequence call 1
...
N60 ... ;Start number 1
...
N90 ... ;Start number 2
...
N120 ... ;Return number 2
...
N130 L SEQUENCE [N90 N120 REPEAT=4] ;Sequence call 2
...
N150 ... ;Return number 1
...
Repeat block sequence between 2 block numbers in a global subroutine several times:
...
N20 ...
...
N80 L SEQUENCE [NAME="glob_1.nc" N50 N150 REPEAT=4]
...
Nested multiple calls of block sequences in the current program and a global subroutine between block numbers:
...
N20 L SEQUENCE [N60 N150 REPEAT=2] ;Sequence call 1
...
N60 ... ;Start number 1
...
N80 L SEQUENCE [NAME="glob_1.nc" N50 N150 REPEAT=3] ;Sequence call 2
...
N150 ... ;Return number 1
...
Alternatively, a block sequence can also be programmed by jump labels.
Syntax of L SEQUENCE when using jump labels (string labels): |
L SEQUENCE [ [ NAME=<string> ] [<START>] [ [<END>] ] [ REPEAT=.. ] [ ENDTAG ] ] |
NAME=<string> | Name of the current subroutine or a global subroutine in which the block sequence makes a pass. Optional: If no name is programmed, the block sequence passes through the current NC program. |
[<START>] | Start label of first block to be executed (start of block sequence) |
[<END>] | End label of last block to be executed (return, end of block sequence) Optionally, if both block numbers are identical or only the start label is specified, only this block is executed. |
REPEAT=.. | Number of repetitions of a block sequence, positive integer > 1. Optionally, if REPEAT is not specified, the block sequence makes a single pass. |
ENDTAG | Marks the call L SEQUENCE itself as an additional valid end of the block sequence. Optionally, if both ENDTAG and an end label are programmed at the same time, the sequence end found first is the valid one. |
The controller searches for the programmed jump labels in the specified NC program (which can also be the same program that calls the command). The two jump labels mark the first and last NC blocks to be executed in the block sequence; NC blocks outside this block sequence are not executed.
Jump labels are set at block start or directly after the block number. An error message is issued if the start or return label is not found.
If the block sequence is to be executed multiple times (REPEAT > 1), the program starts at the start label again when it reaches the end of the block sequence. Once all passes have been executed, the program returns from the block sequence and the rest of the program sequence is continued.
If only a single start label was specified in the command, only this line is passed. This corresponds to a call with two identical N numbers.
The L SEQUENCE call itself may be located in the block sequence defined by the jump labels. When the same call is read again, the following two reactions are possible:
Without ENDTAG the recall is ignored and the block sequence is executed up to the return label.
With ENDTAG the L SEQUENCE call is marked as the valid sequence end and the block sequence is terminated.
Programing Example
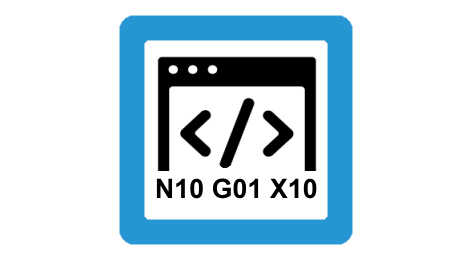
Calling block sequences with jump labels (L SEQUENCE)
Repeat block sequence between 2 jump labels once:
...
N20 [STARTLBL] ... ;Start label
...
N50 [ENDLBL] ... ;Return label
...
N80 L SEQUENCE [[STARTLBL] [ENDLBL]] ;or..
N80 L SEQUENCE [[STARTLBL] [ENDLBL] REPEAT=1]
...
Repeat block sequence between 2 jump labels several times:
...
N20 [STARTLBL] ... ;Start label
...
N50 [ENDLBL] ... ;Return label
...
N80 L SEQUENCE [[STARTLBL] [ENDLBL] REPEAT=4]
...
Block sequence between 2 jump labels. Bracketed sequence call is ignored when the block sequence is executed since ENDTAG is not set:
...
N20 [STARTLBL] ... ;Start label
...
N40 L SEQUENCE [[STARTLBL] [ENDLBL]]
...
N80 [ENDLBL]... ;Return label
...
Block sequence between 2 jump labels. Bracketed sequence call is the first block sequence end found since ENDTAG is set:
...
N20 [STARTLBL]... ;Start label
...
N40 L SEQUENCE [[STARTLBL] [ENDLBL] ENDTAG]
...
N80 [ENDLBL] ... ;Return label
...
Block sequence between 2 jump labels with ENDTAG. ENDTAG is not relevant since the return label is before the sequence call:
...
N20 [STARTLBL]... ;Start label
...
N50 [ENDLBL] ... ;Return label
...
N80 L SEQUENCE [[STARTLBL] [ENDLBL] REPEAT=4 ENDTAG]
...
Repeat a single NC block several times:
...
N20 [STARTLBL] ... ;Start label
...
N80 L SEQUENCE [[STARTLBL] REPEAT=4] ;or..
N80 L SEQUENCE [[STARTLBL] [STARTLBL] REPEAT=4]
...
Block sequence between 1 jump label and ENDTAG:
...
N20 [STARTLBL] ... ;Start label
...
N80 L SEQUENCE [[STARTLBL] ENDTAG]
...
Repeat block sequence between 2 jump labels several times. Sequence call is before the block sequence:
...
N80 L SEQUENCE [[STARTLBL] [ENDLBL] REPEAT=4]
...
N100 [STARTLBL] ... ;Start label
...
N150 [ENDLBL] ... ;Return label
Nested multiple call of block sequences between jump labels:
...
N40 L SEQUENCE [[STARTLBL1] [ENDLBL1] REPEAT=2] ;Sequence call 1
...
N60 [STARTLBL1] ... ;Start label 1
...
N90 [STARTLBL2] ... ;Start label 2
...
N120 [ENDLBL2] ... ;Return label 2
...
N130 L SEQUENCE [[STARTLBL2] [ENDLBL2] REPEAT=4] ;Sequence call 2
...
N150 [ENDLBL1]... ;Return label 1
...
Repeat block sequence between 2 jump labels in a global subroutine several times:
...
N20 ...
...
N80 L SEQUENCE [NAME="glob_1.nc" [SUP1] [EUP1] REPEAT=4]
...
Nested multiple calls of block sequences in the current program and a global subroutine between jump labels:
...
N20 L SEQUENCE [[STARTLBL] [ENDLBL] REPEAT=2] ;Sequence call 1
...
N60 [STARTLBL] ... ;Start label 1
...
N80 L SEQUENCE [NAME="glob_1.nc" [SUP1] [EUP1] REPEAT=3] ;Sequence call 2
...
N150 [ENDLBL]... ;Return label 1
...
When programming with block numbers, a block sequence is terminated by specifying the return number; when programming with jump labels a block sequence is terminated by specifying the end label or by specifying the keyword ENDTAG in the call L SEQUENCE itself.
Alternatively, a block sequence can also be terminated by specifying the NC command #SEQUENCE END.
Syntax of default end label: |
#SEQUENCE END |
Notice
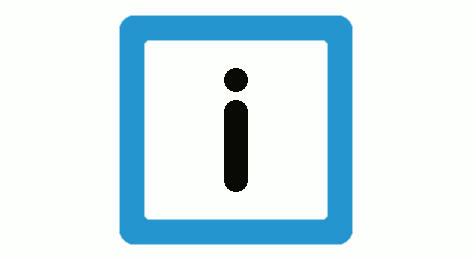
No other NC commands may be programmed in the same NC block together with #SEQUENCE END, with the exception of a block number.
Then it is no longer necessary to specify an end label in the L SEQUENCE call. Only the start label must be set together with the additional information that this start label does not address a single NC block but the start of a block sequence. The program name, number of repetitions and ENDTAG are also optional.
Syntax of L SEQUENCE with block number or jump label in combination with #SEQUENCE END: |
L SEQUENCE [ [ NAME=<string> ] N.. | [<START>] BEGIN [ REPEAT=.. ] [ ENDTAG ] ] |
NAME=<string> | Name of the current subroutine or a global subroutine in which the block sequence makes a pass. Optional: If no name is programmed, the block sequence passes through the current NC program. |
N.. | Number of the first block to be executed (start of block sequence) |
[<START>] | Start label of first block to be executed (start of block sequence) |
BEGIN | Start number or start label addresses the start of a block sequence. #SEQUENCE END or ENDTAG mark the sequence end. |
REPEAT=.. | Number of repetitions of a block sequence, positive integer > 1. Optionally, if REPEAT is not specified, the block sequence makes a single pass. |
ENDTAG | Marks the call L SEQUENCE itself as an additional valid end of the block sequence. Optionally, if both ENDTAG and #SEQUENCE END are specified at the same time, the sequence end found first is the valid one. |
Programing Example
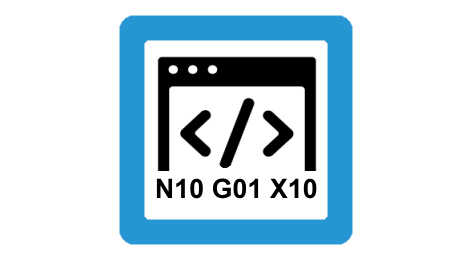
Calling block sequences (L SEQUENCE) with end label #SEQUENCE END
Execute block sequence between start number and end label once.
...
N20 ... ;Start number
...
N50 #SEQUENCE END ;End label
...
N80 L SEQUENCE [N20 BEGIN]
...
Repeat block sequence between start label and end label multiple times:
...
N20 [STARTLBL]... ;Start label
...
N50 #SEQUENCE END ;End label
...
N80 L SEQUENCE [[STARTLBL] BEGIN REPEAT=4]
...
Execute block sequence between start label and end label once. Sequence call is before the block sequence:
...
N80 L SEQUENCE [[STARTLBL] BEGIN]
...
N100 [STARTLBL] ... ;Start label
...
N150 #SEQUENCE END ;End label
Repeat block sequence between start number and end label in a global subroutine multiple times:
...
N80 L SEQUENCE [NAME="glob_1.nc" N50 BEGIN REPEAT=4]
...
Block sequence between start label and end label. Bracketed sequence call is the first block sequence end found since ENDTAG is set:
...
N20 [STARTLBL]... ;Start label
...
N40 L SEQUENCE [[STARTLBL] BEGIN ENDTAG]
...
N80 #SEQUENCE END ;End label
...