Operations for character strings
Overview of all available operations:
String operations:
Adding strings | The + character combines 2 strings. V.E.str = "Hello" + " world!" (-> Result is "Hello world!") |
+ |
|
Determine | LEFT supplies the left starting string of a string. Get anz characters from string str based on the first character. V.E.str = LEFT["Hello world!", 5] (-> Result is "Hello") |
LEFT[str, anz] |
|
Determine | MID supplies the substring of a string. Get anz characters from string str starting with character at position pos. V.E.str = MID["How are you?", 3, 5] (-> Result is "are") |
MID[str, anz, pos] |
Determine | RIGHT supplies the right final string of a string. Get anz characters from string str based on the final character. V.E.str = RIGHT["Hello world! How are you?", 12] (-> Result is "How are you?") |
RIGHT[str, anz] |
Determining string length | LEN determines the length (number of characters) of a string. P1 = LEN["Hello world! How are you?"] (-> Result is 25) |
LEN[str] |
Determine character value | ORD supplies the numerical value of a character in a string at position pos. P1 = ORD["Hello world!”, 1] (-> Result is 72, character value of "H”) P2 = ORD["Hello world!”, 7] (-> Result is 119, character value of "w”) If no position pos is specified, the value of the first character is returned. If a position pos greater than the length of the character string is specified, the error ID 21545 is output. For ASCII characters, the ORD function returns the exact ASCII value. The return value for empty character strings is 0. |
ORD[str, pos] |
Notice
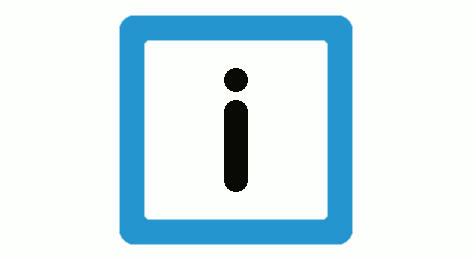
FIND[..] is case-sensitive (uppercase/lowercase).
Search for substring | FIND searches for a string str2 in a string str1 and gives the position of the first match of str2 in str1. V.E.str1 = "Hello world! How are you?" V.E.str2 = "How" P1 = FIND[V.E.str1, V.E.str2] (-> Result is 14) If string str2 in string str1 does not exist, FIND gives the result 0. V.E.str1 = "Hello world! How are you?" V.E.str2 = "today" P1 = FIND[V.E.str1, V.E.str2] (-> Result is 0) |
FIND[str1, str2] |
Deleting a substring | DELETE deletes in string str a specific number of characters anz, starting with the character at position pos. V.E.str = DELETE["Hello world! How are you?", 5, 7] (-> Result is "Hello ! How are you?") |
DELETE[str, anz, pos] |
Inserting a substring | INSERT inserts string str2 in string str1 starting after the character at position pos. V.E.str1 = "Hello ! How are you?" V.E.str2 = "world" V.E.str = INSERT[V.E.str1, V.E.str2, 6] (-> Result is "Hello world! How are you?") |
INSERT[str1, str2, pos] |
Replacing a substring | REPLACE replaces a number of characters anz in string str1 by the substring str2, starting with the character at position pos. V.E.str1 = "What is your name?" V.E.str2 = "age" V.E.str = REPLACE[V.E.str1, V.E.str2, 4, 14] (-> Result is "What is your age?") |
REPLACE[str1, str2, anz, pos] |
Combining strings | FSTRING generates strings with a dynamic content. In principle, the number of substrings in unlimited and consists of statis and dynamic elements.
The result of an FSTRING operation is a string. It can also be used to continue the calculation with a plus (or other operators permitted for strings).
This also permits the nesting of FSTRINGs:
V.L.Number = 123 V.L.Float = 3.57 V.E.str = FSTRING["V.L.Number: ", V.L.Number, (-> Result is: "V.L.Number: 123 / V.L.Float: 3.57") |
FSTRING["str_stat", str_dyn, {"str_stat", str_dyn,}] |
Comparison operators:
Notice
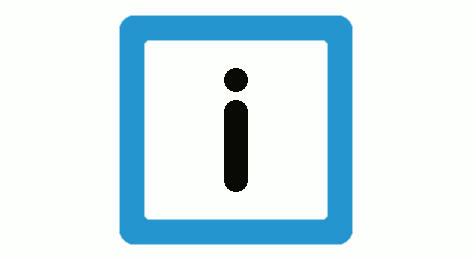
Comparison operations are case-sensitive.
Equality | V.E.str1 = "Peter" V.E.str2 = "Peter" $IF V.E.str1 == V.E.str2 #MSG ["%s is equal to %s!", V.E.str1, V.E.str2] $ELSE #MSG ["Strings are not equal!"] $ENDIF (-> Result is "Peter is equal to Peter") |
== |
Inequality | V.E.str1 = "Peter" V.E.str2 = "Steve" $IF V.E.str1 != V.E.str2 #MSG ["%s is not equal to %s!", V.E.str1, V.E.str2] $ELSE #MSG ["Strings are equal!"] $ENDIF (-> Result is "Peter is not equal to Steve") |
!= |
Greater than or Greater than or equal to | V.E.str1 = "Peter" V.E.str2 = "Peter" $IF V.E.str1 > V.E.str2 #MSG ["%s is greater than or equal to %s!", V.E.str1, V.E.str2] $ELSEIF V.E.str1 >= V.E.str2 #MSG ["%s is greater than or equal to %s!", V.E.str1, V.E.str2] $ENDIF (-> Result is "Peter is greater than or equal to Peter!") V.E.str1 = "Peter" V.E.str2 = "Bob" $IF V.E.str1 > V.E.str2 #MSG ["%s is greater than or equal to %s!", V.E.str1, V.E.str2] $ELSEIF V.E.str1 >= V.E.str2 #MSG ["%s is greater than or equal to %s!", V.E.str1, V.E.str2] $ENDIF (-> Result is "Peter is greater than Bob!") |
>
>= |
Less than or Less than or equal to | V.E.str1 = "Peter" V.E.str2 = "Peter" $IF V.E.str1 < V.E.str2 #MSG ["%s is less than %s!", V.E.str1, V.E.str2] $ELSEIF V.E.str1 <= V.E.str2 #MSG ["%s is less than or equal to %s!", V.E.str1, V.E.str2] $ENDIF (-> Result is "Peter is less than or equal to Peter!") V.E.str1 = "Bob" V.E.str2 = "Tim" $IF V.E.str1 < V.E.str2 #MSG ["%s is less than %s!", V.E.str1, V.E.str2] $ELSEIF V.E.str1 <= V.E.str2 #MSG ["%s is less than or equal to %s!", V.E.str1, V.E.str2] $ENDIF (-> Result is "Bob is less than Tim!") |
<
<= |
Conversion functions:
Integer to String | INT_TO_STR[...] | V.E.str = INT_TO_STR[123] |
Real to String | REAL_TO_STR[...] | V.E.str = REAL_TO_STR[12.34] |
String to Integer | STR_TO_INT[...] | V.E.sgn32 = STR_TO_INT["12"] |
String to Real | STR_TO_REAL[...] | V.E.real64 = STR_TO_REAL["123.45"] |