Arithmetic expressions <expr>
The usual calculation rules apply to handling arithmetic expressions:
- order-of-operations rule
- the parenthesis rule, whereby square brackets "[ ]" must be used. Round parenthesis "(...)” are for comments.
Parameters are often used in arithmetic expressions. The notation of parameters is:
- P followed by an integer, e.g. P12.
Example of an arithmetic expression:
P5 = [[sin[R1*30.00] + P2] / P5]
Macros (strings) may be assigned to arithmetical expressions and parts of them.
A macro name leads to a macro content which is analysed. Recursive handling is also possible.
Macro names must be placed in quotation marks. When decoded, the notation is case-sensitive (uppercase/lowercase).
Nested strings are identified by a preceding '\' before the double quotation marks. Make sure that complete nesting levels are always grouped in a macro, i.e. adding ´[´ at the start and ´]´ at the end of macro content should have no effect on the result of the mathematical expression.
Programing Example
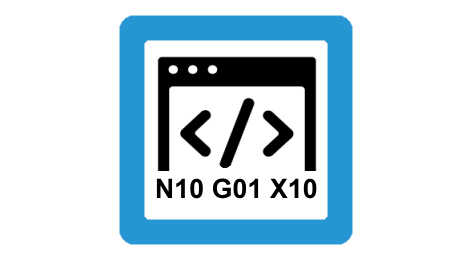
Nested macros
Correct:
N10 "STRING1" = "COS[\"STRING2\"]"
N20 "STRING2" = "5 * 12"
N30 "STRING3" = "SIN[89.5 + \"STRING1\"]"
N40 X[-2 * "STRING1" + "STRING2" + "STRING3"] (Move to X60)
M30
Wrong:
Only complete nesting levels may be compiled
in the string.
N10 "STRING1" = "COS["
N20 "STRING2" = "90]"
N30 "STRING3" = " \"STRING1\" \"STRING2\" "
Macros defined in the NC program are valid program global.
Section "Macroprogramming (# INIT MACRO TAB) “ describes how to program macros outside mathematical expressions.
Overview of all available calculation operations:
Basic types of calculation:
Addition | + | P1 = P2 + P3 + 0.357 |
Subtraction | - | P1 = P2 - 0.031 |
Multiplication | * | P1 = P2 * [P3 + 0.5] |
Division | / | P1 = P2 * P3 / [P5 + P6] |
Exponential calculation | ** | P1 = 2**P3 (2 to the power P3) |
Modulo calculation | MOD | P1 = 11 MOD 3 (-> 2) |
Numerical functions:
Absolute value formation | ABS [..] | P1 = ABS [P2 - P4] |
Squaring | SQR [..] | P1 = SQR [P2] + SQR [P3] |
Square root | SQRT [..] | P1 = SQRT [SQR[P2]+SQR[P3]] |
e function | EXP [..] | P1 = EXP [P2 * P4] |
Natural logarithm | LN [..] | P1 = LN [P2] + LN [P3] |
To the power of ten | DEXP [..] | P1 = DEXP [P2] |
Common logarithm | LOG [..] | P1 = LOG [P2] |
Attention
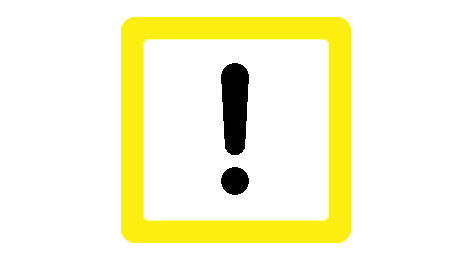
In the case of LN, LOG and SQRT the argument must always be greater than 0!
Bit operators:
AND operation | & | P1 = P2 & P3 |
OR operation | | | P1 = P2 | P3 |
Exclusive OR | ^ | P1 = P2 ^ P3 |
Complement | INV[..] | P1 = INV[P2] |
Attention
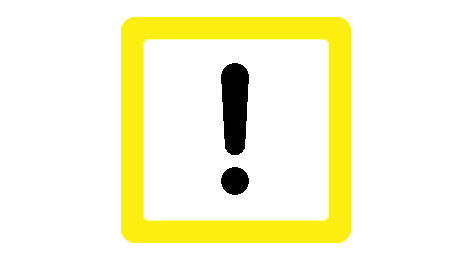
The operands may be any positive mathematical expression or number within the range 0 ... 2^32-1 (UNS32). Negative expressions or numerals are not allowed. Floating point numbers are converted into integers.
The result of a bit operation is always within the range of 0... 2^32-1 (UNS32).
Logic operators:
AND operation | && / AND | P1 = P2 && P3 P1 = P2 AND P3 |
OR operation | || / OR | P1 = P2 || P3 P1 = P2 OR P3 |
Exclusive OR operation | XOR | P1 = P2 XOR P3 |
NOT operation | NOT[..] | P1 = NOT[P2] P1 = NOT[1] (P1 = 0) P1 = NOT[0.5] (P1 = 0) P1 = NOT[0.49] (P1 = 1) P1 = NOT[0] (P1 = 1) |
Attention
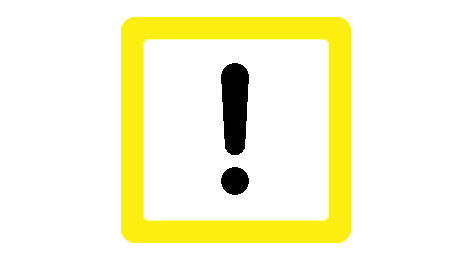
Operands may be any positive mathematical expression or numeral. Negative expressions or numerals are not allowed.
A floating point numeral is evaluated as TRUE (1) if its value is > or = 0.5.
Comparison operators:
Loops (Section Statements for influencing NC program flow) require comparison expressions. Verification can be conducted as follows:
Equality | == | $IF P1 == 10 |
Inequality | != | $IF P1 != 10 |
Greater than or equal to | >= | $IF P1 >= 10 |
Less than or equal to | <= | $IF P1 <= 10 |
Less than | < | $IF P1 < 10 |
Greater than | > | $IF P1 > 10 |
Operator priorities:
The priorities of available operators are listed in descending order. 10 is the highest and 1 is the lowest priority.
Priority | Operator | Description |
10 | ** | Power |
9 | *, / | Multiplication, division |
8 | +, - | Addition, subtraction |
7 | & | Bitwise AND |
6 | ^ | Bitwise exclusive OR |
5 | | | Bitwise OR |
4 | <=, >=, ==, <, >, != | Comparison operators |
3 | &&, AND | Logic AND |
2 | XOR | Logic exclusive OR |
1 | ||, OR | Logic OR |
Possible truth values are:
True | TRUE | $IF V.A.MERF.X == TRUE |
Not true | FALSE | $WHILE V.G.WZ[2].OK == FALSE |
Attention
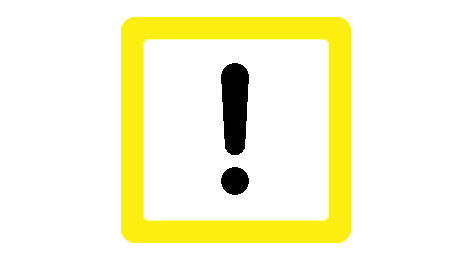
Processing truth values:
For TRUE, the value 1 is used in the controller.
For FALSE, the value 0 is used in the controller.
Trigonometric functions (angles specified in degrees):
Sine | SIN [..] | P1 = SIN [P2] |
Cosine | COS [..] | P1 = COS [P2] |
Tangent | TAN [..] | P1 = TAN [P2] |
Cotangent | COT [..] | P1 = COT [P2] |
Arcsine | ASIN [..] | P1 = ASIN [P2] |
Arccosine | ACOS [..] | P1 = ACOS [P2] |
Arctangent | ATAN [..] | P1 = ATAN [P2] |
Arctangent with | ATAN2 [y,x] | P1 = ATAN2[100,100] (-> result is 45°) |
Arc cotangent | ACOT [..] | P1 = ACOT [P2] |
Attention
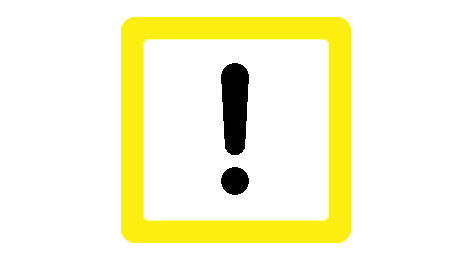
With the numerical functions ASIN and ACOS, the argument must always be between -1 and +1.
For the numerical function TAN, the argument should not assume the values... -90, 90, 270 ... degrees.
For the numerical function COT, the argument should not assume the values... -180, 0, 180 ... degrees.
The numerical function ATAN2 results in x!=0 for the angle of a position relative to the X axis in the correct quadrant.
Special case: For ATAN2[0.0] (x = 0 and y = 0), the result is always 0.
Transformation functions:
Remove | INT [..] | P1 = INT [123,567] (P1 = 123) |
Remove | FRACT [..] | P1 = FRACT [123,567] (P1 = 0,567) |
Round up to integer | ROUND [..] | P1 = ROUND [77.5] (P1 = 78) P1 = ROUND [45.4] (P1 = 45) |
Round up | CEIL [..] | P1 = CEIL [8.3] (P1 = 9) |
Round down | FLOOR [..] | P1 = FLOOR [8.7] (P1 = 8) |
Constants:
3.141592654 (π) | PI | P2 = 2*PI (P2 = 6.283185307) |
Special functions:
Check for existence of variables (V.P., V.L., V.S., V.E.) / parameters / M/H functions / macros |
EXIST [<Variable/ Parameter/ M function/ H function/ macro_name>] | $IF EXIST[V.P.MYVAR] == TRUE $IF EXIST[V.L.MYARRAY[0]] == TRUE * $IF EXIST[P1] != TRUE $IF EXIST[M55] == TRUE $IF EXIST[H20] == TRUE $IF EXIST["Macro1"] == TRUE *For arrays with valid indices! |
Determining the size of an array dimension of variables (V.P., V.L., V.S., V.E.) / parameters |
SIZEOF [<Array_name>, <Dimension>]
or for 1. Dim. SIZEOF [<Array_name>] | #VAR P99[3][4]= [1,2,3,4, #ENDVAR (P12 and P13 for 1st dimension) P12 = SIZEOF[P99] (P12 = 3) P13 = SIZEOF[P99,1] (P13 ==P12 =3) (P14 for 2nd dimension) P14 = SIZEOF[P99,2] (P14 = 4) (P15 for 3rd dimension that does not exist) P15 = SIZEOF[P99,3] (P15= -1) SIZEOF always results in -1 for non-existent array dimensions and for variables that are not arrays. |
Determine greater value | MIN [x,y] | P1 = MIN [P2, P3] |
Determine greater value | MAX [x,y] | P1 = MAX [P2, P3] |
Determine sign | SIGN [..] | P1 = SIGN [P2] results in positive values: 1 negative values: -1 Zero: 0 |
Determining the string length of a macro content. [as of V2.11.2841.00] | MACRO_LENGTH [<macro_name>] | "Macro53" = "G53 X0 Y0 Z0" "Empty" = "" P1 = MACRO_LENGTH["Macro53"] P1 = MACRO_LENGTH["Empty"] MACRO_LENGTH always results in -1 for non-existent macros. |
Reading and resolving a macro content. The return value is a string. [as of V3.1.3081.4] | <MACRO_CONTENT[<macro_name>] | "MACRO_1" = "1 + 2" "MACRO_2" = "SIN[\"MACRO_1\"]" MACRO_CONTENT["MACRO_1"] returns "1 + 2" MACRO_CONTENT["MACRO_2"] returns "SIN[1 + 2]" |
Encryption function:
This function is used to encrypt strings. The related key is user-definable. Strings may contain important data that require protection by encryption.
Encrypted data can then be saved to file with #MSG SAVE, for example, or supplied to the PLC by V.E. variables.
Encrypt string | ENCRYPT ["key", "string"] |
Notice
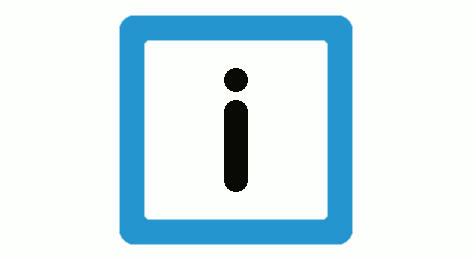
Due to an EU export regulation, the decryption function is no longer available.
The product of ENCRYPT is assigned to a string-type variable. In this case, note the following:
- The string variable must at least be double the length of the encrypted string.
Programing Example
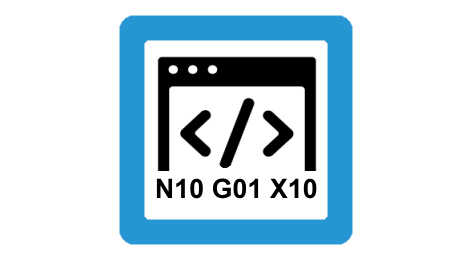
Encrypt string and save to file
N10 V.E.encrypted = ENCRYPT[ "Key", "Encrypted string" ]
N20 #MSG SAVE ["Encrypted text = %s", V.E.encrypted ]
..
M30